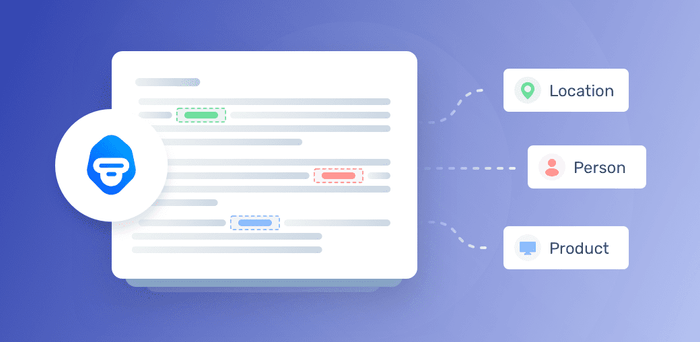
Named Entity Recognition with BERT
Named Entity Recognition with BERT utilizes cutting-edge technology to accurately identify and categorize named entities in textual data. By leveraging BERT's advanced capabilities, this tool streamlines information extraction processes by recognizing entities like names of individuals, organizations, and locations within text, enhancing text analysis efficiency.
mit
Token Classification
PyTorch
Transformers
TensorFlow
Total downloads
5
Created: May 24, 2024