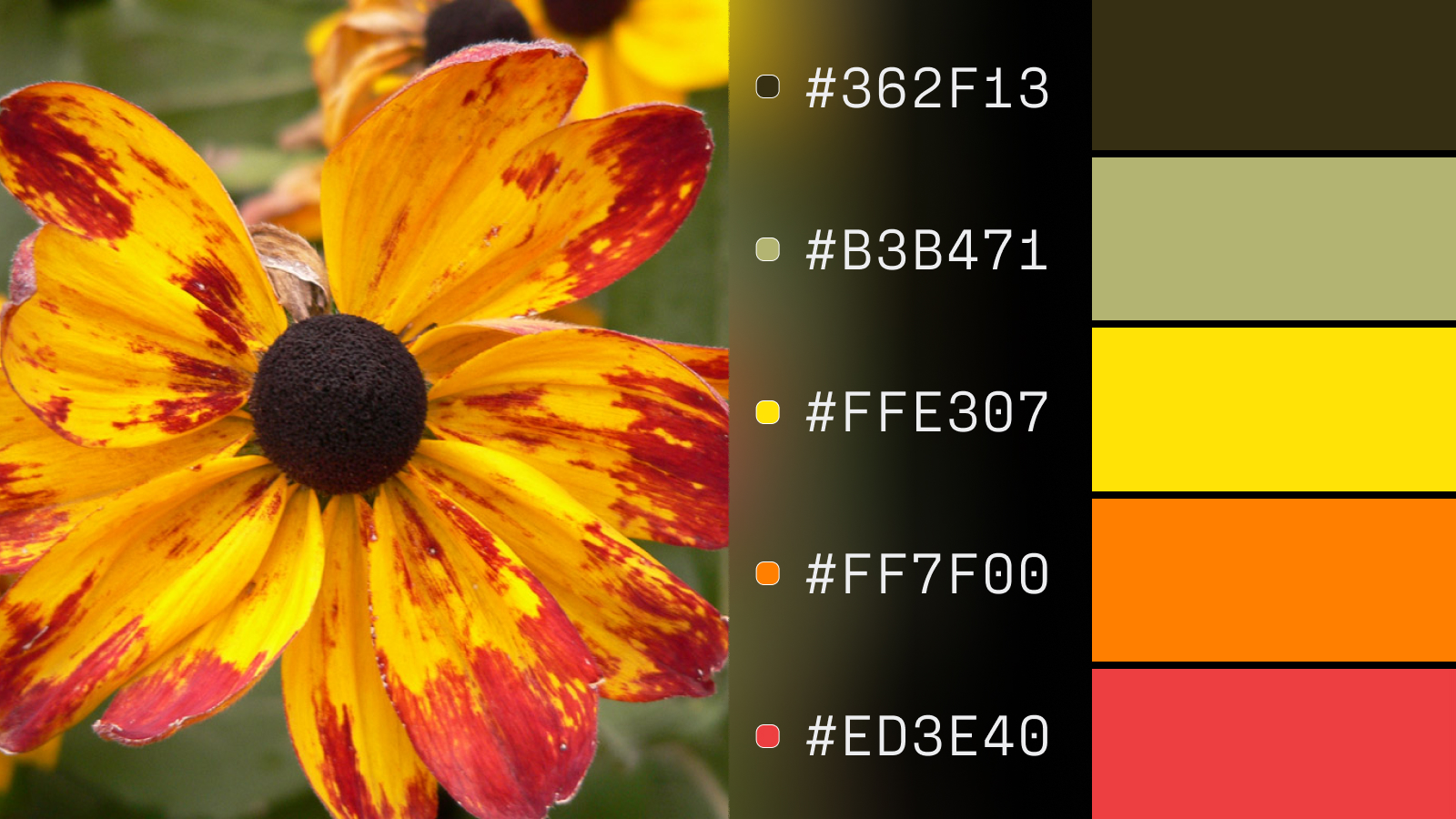
Color Extraction
Color Extraction is a task in computer vision that involves the extraction and analysis of colors from images or videos. The objective of this task is to identify and isolate specific colors or color ranges present in the visual data.
Color Extraction
Summary
Introduction
Color extraction refers to the process of identifying and extracting the prominent colors from an image. It involves analyzing the pixel values of an image to determine the dominant colors present and their corresponding intensities. Color extraction can be useful in various applications, including image analysis, design, visualization, and data representation.
Parameters
Inputs
- input - (image -.png|.jpg|.jpeg): This is the original image that the user wants to analyze for colors.
Output
- output - (image -.png): This is the result of the color analysis process from the original image. It is an image that has been processed to only include important or distinctive colors extracted from the original image.
- code_color - (text): This is the information about the color codes corresponding to the analyzed colors from the original image. It is represented by HEX codes (e.g., #FF0000 for red).
Examples
input | code_color | output |
---|---|---|
![]() | #de7715, #ae2d17, #63623a, #362f13, #f7b808 | ![]() |
![]() | #244011, #e3ce54, #5c5a3b, #debb0b, #867a69 | ![]() |
Usage for developers
Please find below the details to track the information and access the code for processing the model on our platform.
Requirements
python~=3.10
torch~=2.0.0
sklearn
opencv-python
collections
numpy
Pillow
Code based on AIOZ structure
from PIL import Image
from sklearn.cluster import KMeans
from collections import Counter
import numpy as np
import cv2
...
def get_image(pil_image):
...
def get_labels(rimg):
...
def RGB2HEX(color):
...
def do_ai_task(
input: Union[str, Path],
model_storage_directory: Union[str, Path],
device: Literal["cpu", "cuda", "gpu"] = "cpu",
*args, **kwargs) -> Any:
img = get_image(input)
reshaped_img = img.reshape(img.shape[0]*img.shape[1], img.shape[2])
labels, clf = get_labels(reshaped_img)
counts = Counter(labels)
center_colors = clf.cluster_centers_
# We get ordered colors by iterating through the keys
ordered_colors = [center_colors[i] for i in counts.keys()]
hex_colors = [RGB2HEX(ordered_colors[i]) for i in counts.keys()]
width = 100
height = 500
image = Image.new('RGB', (width * len(hex_colors), height))
for i, color in enumerate(hex_colors):
x_start = i * width
x_end = (i + 1) * width
y_start = 0
y_end = height
rgb_color = tuple(int(color.lstrip('#')[index:index+2], 16) for index in (0, 2, 4))
for y in range(y_start, y_end):
for x in range(x_start, x_end):
image.putpixel((x, y), rgb_color)
image.save('output.png')
output = open("output.png", "rb") # io.BufferedReader
return output, hex_colors
Reference
This repository is based on and inspired by Shamima Hossain's work. We sincerely thank them for sharing the code.
License
We respect and comply with the terms of the author's license cited in the Reference section.